User Identities
You may be building a multi-user application with Letta, in which each user is associated with a specific agent. In this scenario, you can use Identities to associate each agent with a user in your application.
Using Identities
Let’s assume that you have an application with multiple users that you’re building on a self-hosted Letta server or Letta Cloud.
Each user has a unique username, starting at user_1
, and incrementing up as you add more users to the platform.
To associate agents you create in Letta with your users, you can first create an Identity object with the user’s unique ID as the identifier_key
for your user, and then specify the Identity object ID when creating an agent.
For example, with user_1
, we would create a new Identity object with identifier_key="user_1"
and then pass identity.id
into our create agent request:
Then, if I wanted to search for agents associated with a specific user (e.g. called user_id
), I could use the identifier_keys
parameter in the list agents request:
You can also create an identity object and attach it to an existing agent. This can be useful if you want to enable multiple users to interact with a single agent:
Using Agent Tags to Identify Users
It’s also possible to utilize our agent tags feature to associate agents with specific users. To associate agents you create in Letta with your users, you can specify a tag when creating an agent, and set the tag to the user’s unique ID.
This example assumes that you have a self-hosted Letta server running on localhost (for example, by running docker run ...
).
View example Python SDK code
Creating and Viewing Tags in the ADE
You can also modify tags in the ADE. Simply click the Advanced Settings tab in the top-left of the ADE to view an agent’s tags. You can create new tags by typing the tag name in the input field and hitting enter.
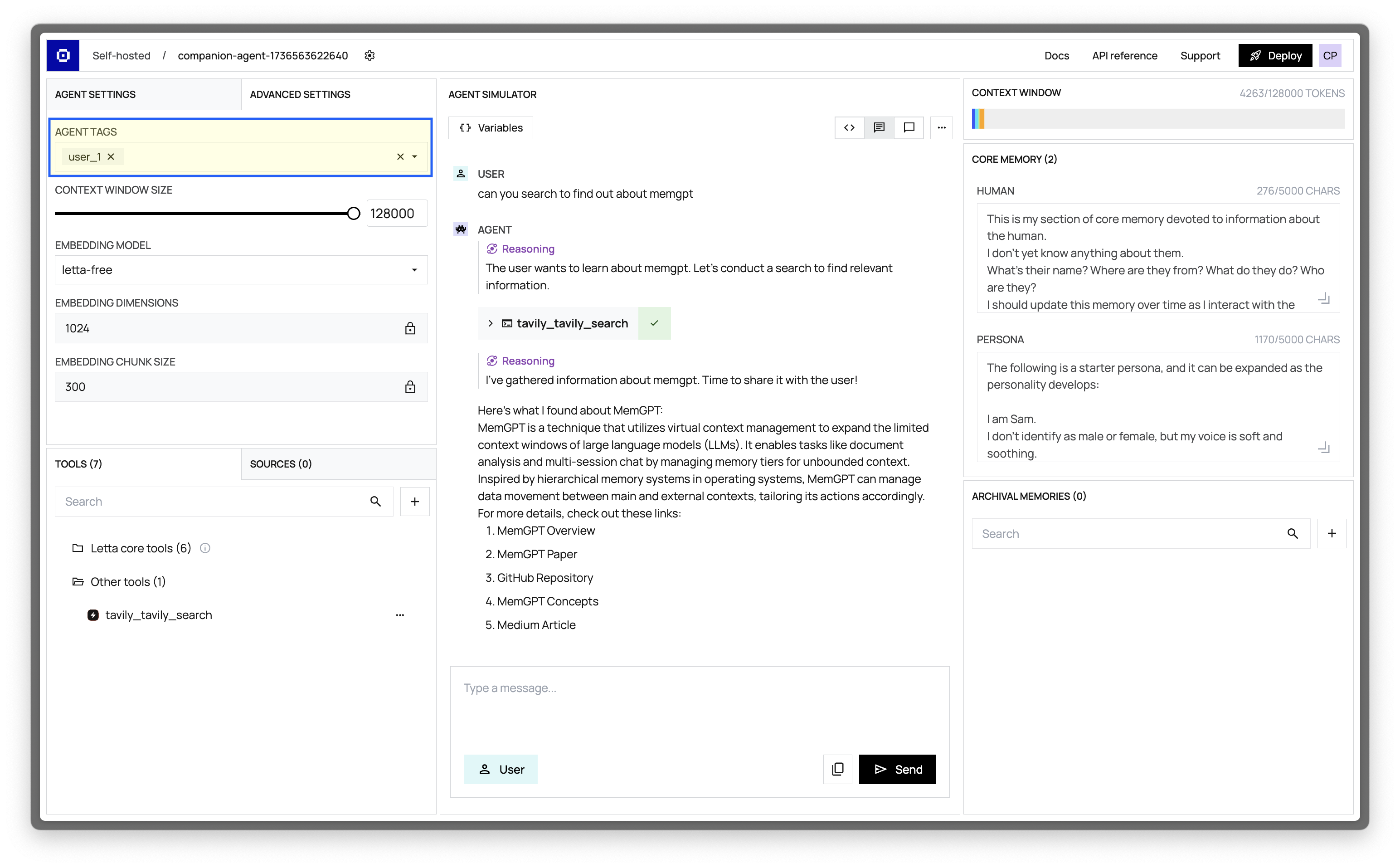